Expressions
JIPipe features its own expression language that is used for generating or filtering statistics or data, dynamically adapting parameters, configuring project or application settings, and more. Thus, it is useful to be familiar with the expression language and associated features.
Expression-style parameters can be easily identified by the term Expression:

Some expressions also indicate when or how many times they are executed:

Each expression parameter comes with an Edit button that opens an editor that lists all available operations, variables, and constants. The editor also applies a syntax check.
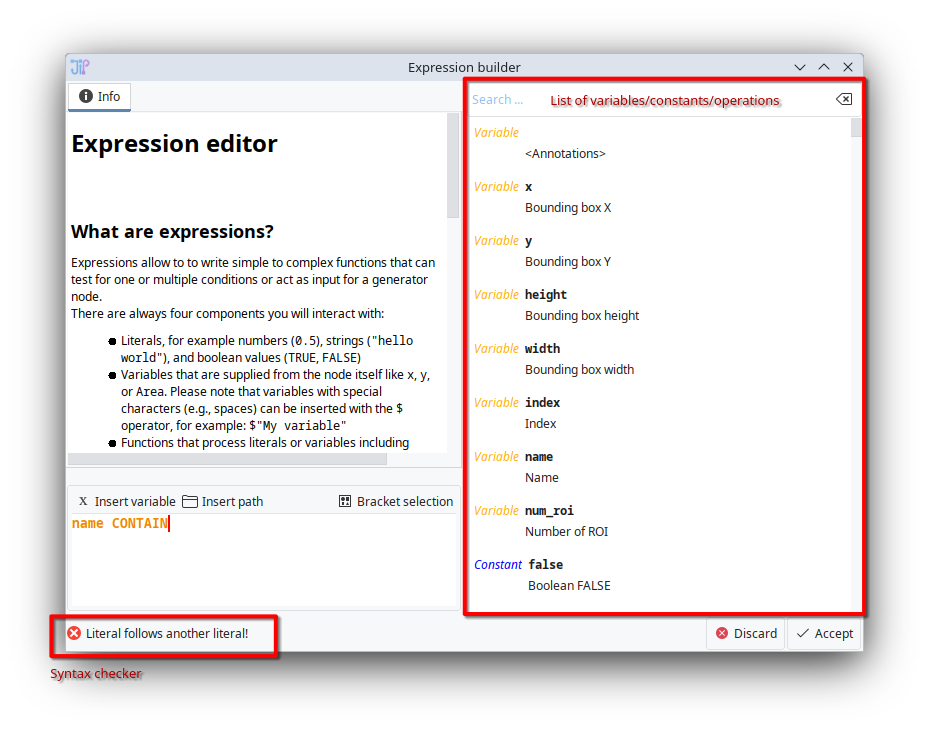
The expression language has six basic components:
- Constants
Predefined values that are useful for a variety of tasks, including e, pi, NaN, or infinity.
- Operators
A variety of operators for processing numbers, text, or lists.
- Functions
Functions to process the variables/constants
- Literals
Quoted strings or numbers
- Variables
Values that are usually received from outside (for example, from a node's workload).
👉 JIPipe identifies anything that it does not know as variable, so typos with constants/operators/functions/literals may yield errors about missing variables.
Empty expressions
Empty expressions automatically fall back to the boolean constant true
, which is derived from the original purpose of expression to be used for data filtering.
Testing expressions
JIPipe provides a developer tool that allows you to play around with expressions similar to a calculator. You can find it by navigating to
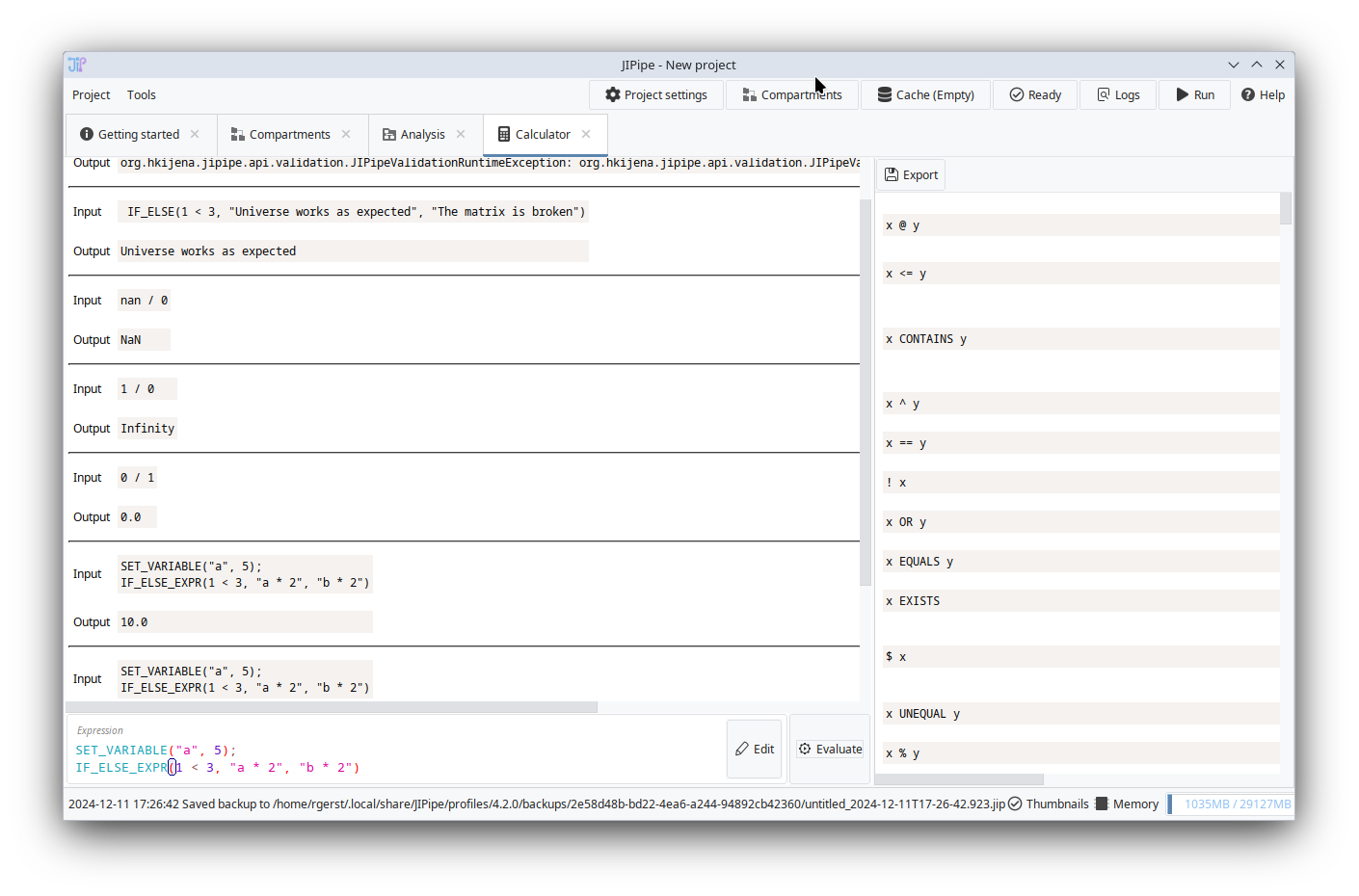
Accessing variables
Variables are usually received from outside and may include annotations, data, measurements, or other values. Most expression-based parameters provide information to the expression editor which variables are available.
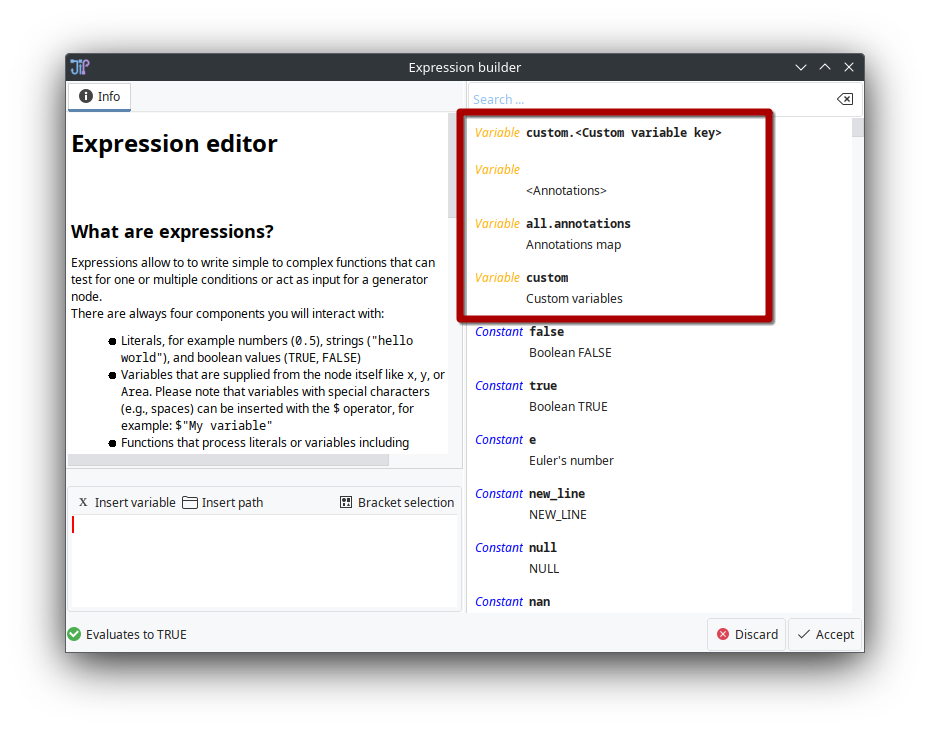
To access a variable within an expression, type it in:
If the variable name contains special reserved characters or spaces, you will have to use the resolve operator $
or GET_VARIABLE
:
Data conversion
Especially when working with text annotations, it is common to convert data into another format. The expression language includes a variety of conversion operations:
- To number
Use
TO_NUMBER
or it's short formNUM
to convert inputs into numbers.NUM("5") // Result is 5.0 NUM(true) // Result is 1.0 NUM(false) // Result is 0.0If you want an integer number, you can also use
TO_INTEGER
TO_INTEGER("5") // Result is 5 TO_INTEGER(true) // Result is 1 TO_INTEGER(false) // Result is 0 TO_INTEGER(5.2) // Result is 5- To boolean
Use
TO_BOOLEAN
to convert data into true/false.For numbers, all values >= 1 are converted to
true
, otherwisefalse
For strings, all values that have a case-insensitve match to
"TRUE"
,"T"
,"ON"
,"YES"
, or"Y"
are converted totrue
Multiple statements
The JIPipe expression language supports multiple statements that are concatenated with the ;
operator. The result of the expression is always the last statement. This is for example useful if you require to set variables within the expression or want to temporarily overwrite the result without deleting the formula.
Example: setting variables
Will return the result of a^2 + a^3
.
Example: temporarily deactivate filter
Will always return true
Quantities
The expression language contains methods to work with quantities (values with units) and allows you to do conversions and extracting information.
Example: Extracting the unit
Will return "microns"
Example: Extracting the value
Will return 1
Example: Unit conversion
Will return "0.001 mm"
Supported units
px, pixels, pixel, nm, µm, microns, micron, mm, cm, dm, m, km, inch, in, foot, ft, yard, yd, ng, µg, mg, g, kg, t, Da, oz, lb, ns, µs, ms, s, min, h, d
Lists/arrays
Expressions come with a variety of operators to work with arrays/lists.
Creating and combining arrays
Arrays are created via the ARRAY
function
Arrays can also be added together:
, which would result in the output [1.0,5.0,8.0,1.0,2.0,3.0]
.
Extracting values
To extract an element from an array, use the @
/[]
operator or GET_ITEM
/GET_ITEM_OR_DEFAULT
:
all return 1.
Checking for item existence
The IN
/CONTAINS
operators can be used to check if an item is contained within an array.
Example: applying a function per array element
Results in [1.0,2.23606797749979,2.8284271247461903]
. The ${ ... } operator is syntactic sugar to avoid having to manually escape more complex functions and can in this case be replaced with "SQRT(value)"
Maps
Expressions can handle map-like structures and come with functions to create maps and extract information.
Creating maps
Maps can be created using the MAP()
function that receives a list of arrays with two items (key and value). For better readability, we recommend to use the PAIR(key, value)
function or the key: value
operator.
All return a map {"a":1.0,"b":2.0}
Checking for key existence
Similar to Python, the IN operator checks the key set of the map:
Extracting values
To extract a value from a map, use the @
/[]
operator or GET_ITEM
/GET_ITEM_OR_DEFAULT
:
JSON
Data can be converted from and to JSON.
Parsing JSON
Use the PARSE_JSON
method to convert JSON data into a map/boolean/array/number:
Creating JSON
Use the TO_JSON
method to convert an object into JSON:
Conditional value (if/else/switch)
The expression language supports the simple conditional processing.
If-else
Use the IF_ELSE
function to return the second or the third parameter depending on the first parameter.
will return "Universe works as expected".
If-else (lazy)
If you know that one of the IF_ELSE
branches will yield an error, you can use IF_ELSE_EXPR
that would receive the branching code as strings.
will return "10" without throwing an error.
Switch-case
A common feature of many programming languages is a function that maps values to other values. This is accessible via the SWITCH_MAP
function.
returns 5.
If the matched value is not present, it stays unchanged.
returns 15.
Switch-case (ladder)
Chaining multiple IF_ELSE
methods can become unreadable. This is why JIPipe provides the SWITCH
and CASE(condition, value)
functions. The surrounding SWITCH
would select the first CASE
where the condition is true
and return its value.
returns "five".
Switch-case (ladder, legacy)
There is an alternative variant to SWITCH
and CASE
called SWITCH_CASE
. Instead of having two functions, there is only one SWITCH_CASE
function where the odd-indexed parameters are mapped to the condition and even-indexed parameters to the values.
Reference: Constants
The expression language defines the following constants:
Name | Value | Description |
---|---|---|
| false | A boolean false value |
| false | A boolean true value |
| 2.718281828459045 | Euler's number |
| "\n" | A new line string (for text) |
| null | Java's null pointer |
| NaN | Floating point (double) NaN |
| -∞ | Floating point (double) negative infinity |
| ∞ | Floating point (double) positive infinity |
| 3.141592653589793 | PI |
| 6.283185307179586 | 2x PI |
Reference: Operators
The following operators are supported, ordered by their precedence (i.e. operators that are higher up are executed before the others)
Precedence | Operator | Supported types | Description | Example |
---|---|---|---|---|
12 |
| Numbers | Negate number |
|
10 |
| Strings | Resolves a variable |
|
10 |
| Numbers | Divides two numbers |
|
9 |
| Lists/Maps/Strings (left), Numbers/Strings (right) | Accesses an element from a list (by index), gets an element from a map, or gets the character at a specific position |
|
7 |
| Strings | Returns true if a variable with the given value exists, otherwise false |
|
7 |
| Numbers | Multiplies two numbers |
|
7 |
| Numbers | Divides two numbers |
|
7 |
| Numbers | Applies modulo division |
|
6 |
| Numbers | Subtract two numbers |
|
6 |
x CONTAINS y
y IN x
| Lists/Strings | Check if a lists/string contains an element/substring |
|
6 |
| Numbers/Strings/Lists | Adds two numbers/lists/strings |
11 + 3
ARRAY(1,2) + ARRAY(3)
"a" + "b" |
5 |
| Numbers | Less than |
|
5 |
| Numbers | Greater than |
|
5 |
| Numbers | Less than or equal |
|
5 |
| Numbers | Greater than or equal |
|
5 | x == y
x EQUALS y | Anything | Equality check |
|
5 |
x != y
x UNEQUAL y
| Anything | Inequality check |
|
3 |
!x
NOT x
| Boolean | Invert boolean |
|
2 |
x & y
x AND y
| Boolean | Boolean AND |
|
2 |
x | y
x OR y
| Boolean | Boolean OR |
|